java pass by value what if reference points to another
Does Coffee laissez passer by reference or pass by value?
Hither's what happens when you laissez passer an object reference to a method in Coffee
Many programming languages allow passing parameters past reference or by value. In Java, we can just pass parameters past value. This imposes some limits and also raises questions. For instance, if the parameter value is changed in the method, what happens to the value following method execution? You may also wonder how Java manages object values in the memory heap. This Java Challenger helps yous resolve these and other common questions about object references in Java.
Object references are passed past value
All object references in Coffee are passed by value. This means that a copy of the value volition be passed to a method. Just the play a joke on is that passing a re-create of the value also changes the real value of the object. To understand why, start with this example:
public form ObjectReferenceExample { public static void primary(String... doYourBest) { Simpson simpson = new Simpson(); transformIntoHomer(simpson); System.out.println(simpson.name); } static void transformIntoHomer(Simpson simpson) { simpson.name = "Homer"; } } form Simpson { String name; }
What do you retrieve the simpson.name
will be after the transformIntoHomer
method is executed?
In this case, it will be Homer! The reason is that Java object variables are simply references that indicate to real objects in the memory heap. Therefore, fifty-fifty though Java passes parameters to methods by value, if the variable points to an object reference, the real object will also be changed.
If you lot're still not quite clear how this works, take a await at the figure below.
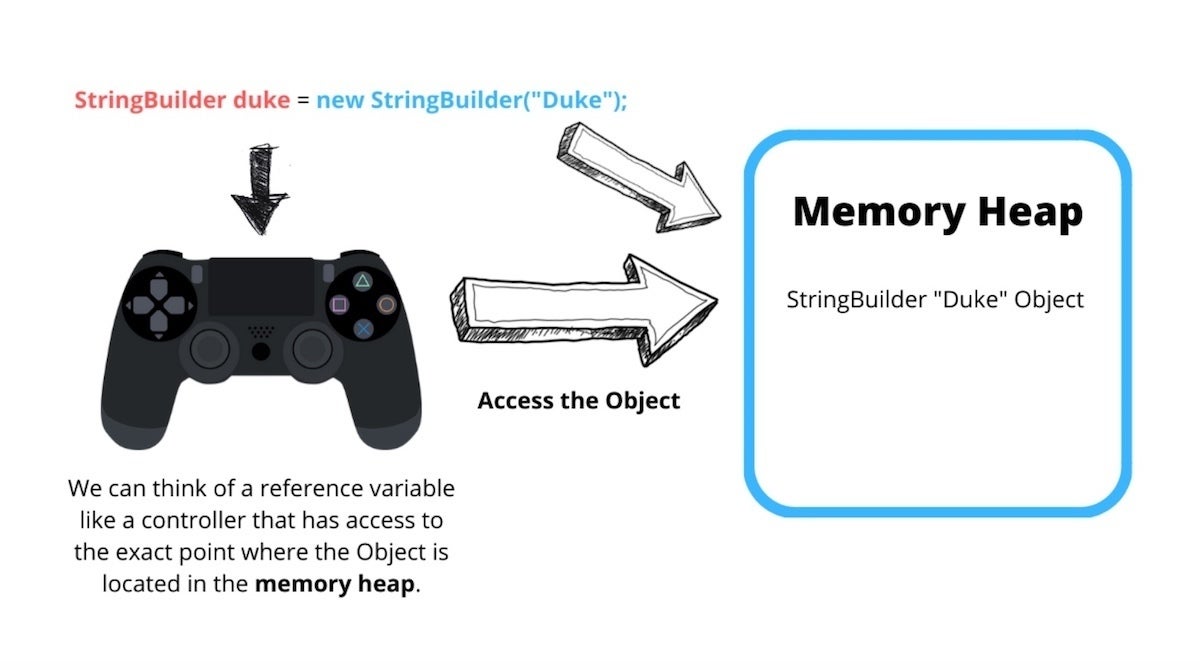
Are primitive types passed by value?
Like object types, primitive types are also passed by value. Can you lot deduce what will happen to the archaic types in the following code example?
public form PrimitiveByValueExample { public static void main(String... primitiveByValue) { int homerAge = xxx; changeHomerAge(homerAge); System.out.println(homerAge); } static void changeHomerAge(int homerAge) { homerAge = 35; } }
If you determined that the value would modify to 30, you are correct. It's xxx because (once again) Java passes object parameters by value. The number 30 is just a copy of the value, not the real value. Primitive types are allocated in the stack retentivity, so only the local value will be changed. In this case, there is no object reference.
Passing immutable object references
What if we did the same test with an immutable String
object?
The JDK contains many immutable classes. Examples include the wrapper types Integer
, Double
, Float
, Long
, Boolean
, BigDecimal
, and of course the very well known String
class.
In the next example, notice what happens when we change the value of a String
.
public class StringValueChange { public static void main(Cord... doYourBest) { String proper name = ""; changeToHomer(name); System.out.println(proper name); } static void changeToHomer(String name) { proper name = "Homer"; } }
What practise you think the output will exist? If y'all guessed "" and so congratulations! That happens because a String
object is immutable, which ways that the fields inside the String
are final and tin't be changed.
Making the Cord
class immutable gives the states ameliorate control over i of Java'southward most used objects. If the value of a String
could be changed, information technology would create a lot of bugs. Also note that nosotros're not changing an attribute of the String
class; instead, nosotros're simply assigning a new Cord
value to information technology. In this example, the "Homer" value will exist passed to name
in the changeToHomer
method. The String
"Homer" will be eligible to exist garbage collected as before long equally the changeToHomer
method completes execution. Even though the object can't exist changed, the local variable volition be.
Passing mutable object references
Unlike String
, nearly objects in the JDK are mutable, like the StringBuilder
class. The case below is similar to the previous 1, but features StringBuilder
rather than String
:
static class MutableObjectReference { public static void main(String... mutableObjectExample) { StringBuilder name = new StringBuilder("Homer "); addSureName(proper noun); System.out.println(name); } static void addSureName(StringBuilder name) { proper name.append("Simpson"); } }
Can you deduce the output for this example? In this instance, because we're working with a mutable object, the output will be "Homer Simpson." You lot could expect the same behaviour from any other mutable object in Java.
You lot've already learned that Java variables are passed by value, meaning that a copy of the value is passed. Only remember that the copied value points to a real object in the Java retention heap. Passing by value nevertheless changes the value of the real object.
Have the object references claiming!
In this Java Challenger nosotros'll examination what you've learned almost object references. In the code example below, you see the immutable String
and the mutable StringBuilder
course. Each is being passed every bit a parameter to a method. Knowing that Java only passes past value, what do you believe will be the output once the master method from this class is executed?
public class DragonWarriorReferenceChallenger { public static void main(Cord... doYourBest) { StringBuilder warriorProfession = new StringBuilder("Dragon "); String warriorWeapon = "Sword "; changeWarriorClass(warriorProfession, warriorWeapon); Organization.out.println("Warrior=" + warriorProfession + " Weapon=" + warriorWeapon); } static void changeWarriorClass(StringBuilder warriorProfession, String weapon) { warriorProfession.append("Knight"); weapon = "Dragon " + weapon; weapon = zip; warriorProfession = null; } }
Here are the options, bank check the end of this article for the answer key.
A: Warrior=null Weapon=zip
B: Warrior=Dragon Weapon=Dragon
C: Warrior=Dragon Knight Weapon=Dragon Sword
D: Warrior=Dragon Knight Weapon=Sword
What'due south simply happened?
The beginning parameter in the higher up instance is the warriorProfession
variable, which is a mutable object. The 2nd parameter, weapon, is an immutable Cord
:
static void changeWarriorClass(StringBuilder warriorProfession, String weapon) { ... }
Now let's analyze what is happening inside this method. At the commencement line of this method, we append the Knight
value to the warriorProfession
variable. Recollect that warriorProfession
is a mutable object; therefore the real object volition be inverse, and the value from it will exist "Dragon Knight."
warriorProfession.append("Knight");
In the second instruction, the immutable local Cord
variable will exist inverse to "Dragon Sword." The real object will never exist changed, however, since String
is immutable and its attributes are terminal:
weapon = "Dragon " + weapon;
Finally, nosotros pass null
to the variables here, only not to the objects. The objects will remain the same equally long as they are still accessible externally--in this instance through the main method. And, although the local variables will be null, aught volition happen to the objects:
weapon = zippo; warriorProfession = null;
From all of this we can conclude that the final values from our mutable StringBuilder
and immutable String
will be:
System.out.println("Warrior=" + warriorProfession + " Weapon=" + warriorWeapon);
The only value that changed in the changeWarriorClass
method was warriorProfession
, because it's a mutable StringBuilder
object. Note that warriorWeapon
did not alter because it's an immutable String
object.
The correct output from our Challenger code would be:
D: Warrior=Dragon Knight Weapon=Sword.
Video claiming! Debugging object references in Java
Debugging is one of the easiest ways to fully absorb programming concepts while also improving your code. In this video you can follow along while I debug and explain object references in Coffee.
Mutual mistakes with object references
- Trying to change an immutable value by reference.
- Trying to change a archaic variable by reference.
- Expecting the real object won't modify when you alter a mutable object parameter in a method.
What to recollect about object references
- Coffee always passes parameter variables by value.
- Object variables in Coffee ever point to the real object in the memory heap.
- A mutable object's value can be changed when information technology is passed to a method.
- An immutable object's value cannot be inverse, even if information technology is passed a new value.
- "Passing by value" refers to passing a re-create of the value.
- "Passing by reference" refers to passing the real reference of the variable in memory.
Learn more than nigh Java
- Get more quick lawmaking tips: Read all of Rafael's posts in the JavaWorld Coffee Challengers serial.
- Acquire more virtually mutable and immutable Java objects (such as
String
andStringBuffer
) and how to use them in your code. - Yous might be surprised to acquire that Java'southward archaic types are controversial. In this characteristic, John I. Moore makes the instance for keeping them, and learning to utilize them well.
- Keep building your Java programming skills in the Java Dev Gym.
- If you lot liked debugging Coffee inheritance, check out more than videos in Rafael'due south Java Challenges video playlist (videos in this series are not affiliated with JavaWorld).
- Want to work on stress free projects and write bug-free lawmaking? Head over to the NoBugsProject for your copy of No Bugs, No Stress - Create Life-Changing Software Without Destroying Your Life.
This story, "Does Java pass past reference or pass by value?" was originally published by JavaWorld .
Copyright © 2020 IDG Communications, Inc.
Source: https://www.infoworld.com/article/3512039/does-java-pass-by-reference-or-pass-by-value.html#:~:text=The%20reason%20is%20that%20Java,object%20will%20also%20be%20changed.
0 Response to "java pass by value what if reference points to another"
Post a Comment